Apple Pay is a payment wallet solution which allows users of Apple devices to make seamless credit/debit card backed payments through a simple and secure 'one-click' user interface. Support for Apple Pay can help improve both conversion and your customer experience, and is rapidly becoming a minimum requirement for proprietary merchant iOS applications that facilitate payment.
Before you start
Accepting Apple Pay payments through your N-Genius Online account relies on a number of pre-requisites, which you must be able to satisfy:
- A valid and enrolled Apple Pay developer account (personal or organization)
- An iOS app integrated with N-Genius Online
You can integrate Apple Pay with N-Genius by the following methods
- Using the N-Genius iOS SDK
- Direct Api Integration
Configuring Apple Pay
In order to begin accepting payments from Apple Pay users, you must first navigate to your N-Genius Online portal, log-in and browse to the Settings > Organisational hierarchy screen, and find the 'Payment channels' configuration option.
On the next screen, you will see a number of tabs indicating each type of payment channel, and how many are already configured.
Switch to the 'Wallets' tab to configure your Apple Pay integration.

Next, click on the 'certificate signing request' link to download the certificate signing request.
Upload this certificate signing request in Apple's developer portal to generate a new Payment processing certificate.
Creating a payment processing certificate
You can navigate to Apple developer portal > Create new Payment processing certificate to create a new payment processing certificate.
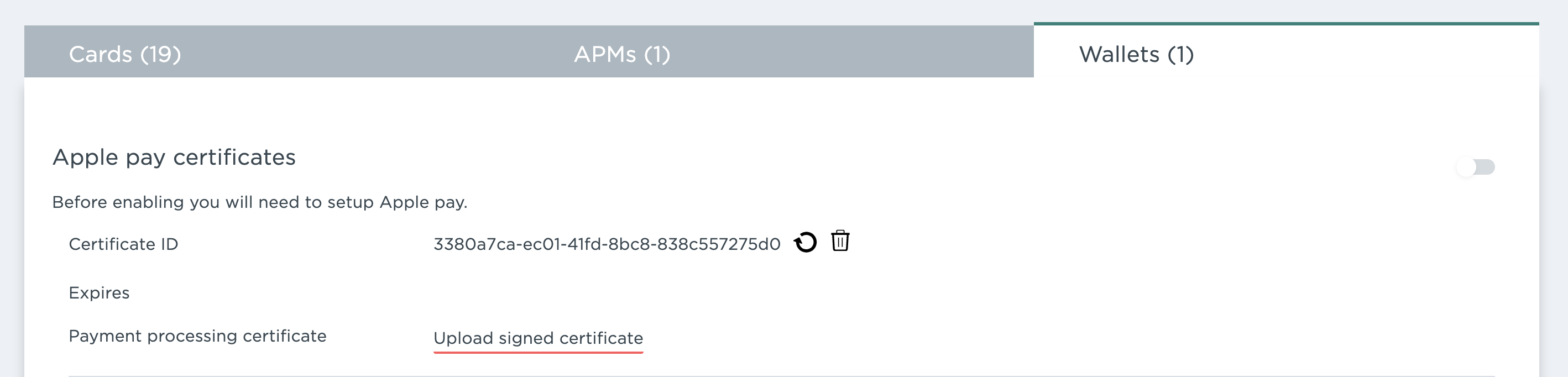
On generating the payment processing certificate (PPC), upload the same on N-Genius portal by clicking the 'Upload signed certificate'.
On uploading the PPC, Apple pay is fully setup on your N-Genius Online account.
Integrating Apple app in-app
There are two ways to setup and configure Apple Pay in your iOS app
- NGenius SDK - Use the sdk to quickly integrate Apple Pay. (Order types supported: "AUTH","SALE")
- Direct API - You can directly integrate using the Apple Pay and NGenius apis to get more control.
1. Enable Apple Pay in-app capabilities
Add your Merchant ID generated in Apple's Developer portal to your project's .entitlements
file under Merchant IDs
2. Implement PKPaymentAuthorizationViewControllerDelegate
PKPaymentAuthorizationViewControllerDelegate
import PassKit
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController,
didSelect paymentMethod: PKPaymentMethod,
handler completion: @escaping (PKPaymentRequestPaymentMethodUpdate) -> Void) {
completion(PKPaymentRequestPaymentMethodUpdate(errors: nil, paymentSummaryItems: paymentSummaryItems))
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController,
didSelect shippingMethod: PKShippingMethod,
handler completion: @escaping (PKPaymentRequestShippingMethodUpdate) -> Void) {
completion(PKPaymentRequestShippingMethodUpdate(paymentSummaryItems: paymentSummaryItems))
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController,
didSelectShippingContact contact: PKContact,
handler completion: @escaping (PKPaymentRequestShippingContactUpdate) -> Void) {
completion(PKPaymentRequestShippingContactUpdate(errors: nil,
paymentSummaryItems: paymentSummaryItems,
shippingMethods: supportedShippingMethods))
}
func paymentAuthorizationViewController(_ controller: PKPaymentAuthorizationViewController,
didAuthorizePayment payment: PKPayment,
handler completion: @escaping (PKPaymentAuthorizationResult) -> Void) {
self.onAuthorizeApplePayCallback(payment, {
authorizationResult, paymentResponse in
DispatchQueue.main.async {
completion(authorizationResult)
controller.dismiss(animated: false, completion: nil)
}
})
}
func paymentAuthorizationViewControllerDidFinish(_ controller: PKPaymentAuthorizationViewController) {
controller.dismiss(animated: false, completion: nil)
}
3. Create the order on Gateway
Please refer the order creation API for creating an order on the gateway. Two stage payments
4. Authorize the transaction on N-Genius
The order created should be authorised to obtain a paymentToken
which is used to further post the Apple Pay encrypted object to N-Genius and process the payment.
The authorization link can be obtained from the order response object. A sample key path is shown below to fetch the payment link
{
"_links": {
"payment-authorization": {
"href": "*https://payment-auth-link*"
}
}
}
A payment token can be requested By making a POST
request to the above link.
-
HTTP Method:
POST
-
Request Headers :
"Accept": "application/vnd.ni-payment.v2+json",
"Media-Type": "application/x-www-form-urlencoded",
"Content-Type": "application/x-www-form-urlencoded"
5. Create the PassKitPaymentRequest Object
This PKPaymentRequest object is required by Apple Pay. You can derive the required fields from the gateway order created. Also care must be taken to populate the merchantIdentifier field with the correct MID field configured in both the Apple's dev portal and N-Genius portal.
The paymentSummaryItems
is an array of PKPaymentSummaryItem
which has information related to the line items of items purchased by the user. This is displayed to the user in the Apple Pay interface. The last Item in this array represents the total cost or the order amount which the customer will be charged.
import PassKit
paymentRequest = PKPaymentRequest()
paymentRequest?.merchantIdentifier = "Insert Mid created in Apple dev portal here"
paymentRequest?.countryCode = "COUNRY CODE"
paymentRequest?.currencyCode = "CURRENCY CODE"
paymentRequest?.requiredShippingContactFields = [.postalAddress, .emailAddress, .phoneNumber]
paymentRequest?.merchantCapabilities = [.capabilityDebit, .capabilityCredit, .capability3DS]
paymentRequest?.requiredBillingContactFields = [.postalAddress, .name]
paymentRequest?.paymentSummaryItems = [PKPaymentSummaryItem(label: "Line Item", amount: NSDecimalNumber(value: amount))]
paymentRequest?.paymentSummaryItems.append(PKPaymentSummaryItem(label: "Total", amount: NSDecimalNumber(value: paymentAmount)))
6. Initiate Apple Pay in app
Initialise the Apple Pay View controller and instantiate it with the PKPaymentRequest object created previously.
import PassKit
let pkPaymentAuthorizationVC = PKPaymentAuthorizationViewController(paymentRequest: applePayRequest)
self.present(pkPaymentAuthorizationVC, animated: false, completion: nil)
7. Post the Apple Pay encrypted object to N-Genius
When the user selects a card from the Apple Pay interface and authorizes a transaction, an encrypted object is received from Apple which needs to be posted to N-Genius in order to process the transaction and charge the customer.
-
HTTP verb :
PUT
-
Body data:
applePayPaymentResponse.token.paymentData
-
Request headers :
"Authorization": "Bearer" + paymentToken,
"Content-Type": "application/vnd.ni-payment.v2+json"
In the above request header, payment token is the token that is received during the authorization step
The body data is the paymentData response received from Apple Pay from the didAuthorizePayment
method of PKPaymentAuthorizationViewControllerDelegate
implemented in step 2
Check if Apple Pay is setup
You can check if a user has setup Apple Pay on his device by making a call to
PKPaymentAuthorizationViewController.canMakePayments()
. This method will return a boolean value true if Apple pay is setup. You can use this method to conditionally render the Apple Pay button.You can also check out Apple's guide to know which devices support Apple Pay
Troubleshooting Apple Pay Issues
While integrating with Apple Pay, if you encounter errors related to decryption or payment failures, this could be an issue of stale certificate being cached by Xcode. In such cases we recommend to create a new merchant identifier (MID) using a string value that has not been previously used in Apple's developer portal and re create the signed certificate against the new MID.
Apple Pay (N-Genius SDK)
Refer the SDK section for details on integrating Apple Pay using the N-Genius iOS SDK.