The N-Genius React Native SDK enables seamless in-app payment acceptance for merchants using React Native. It acts as a wrapper around the native Android and iOS SDKs, allowing cross-platform developers to initiate and manage payments securely.
Before continuing, ensure your backend is set up to authenticate and create orders. Refer to Creating Orders.
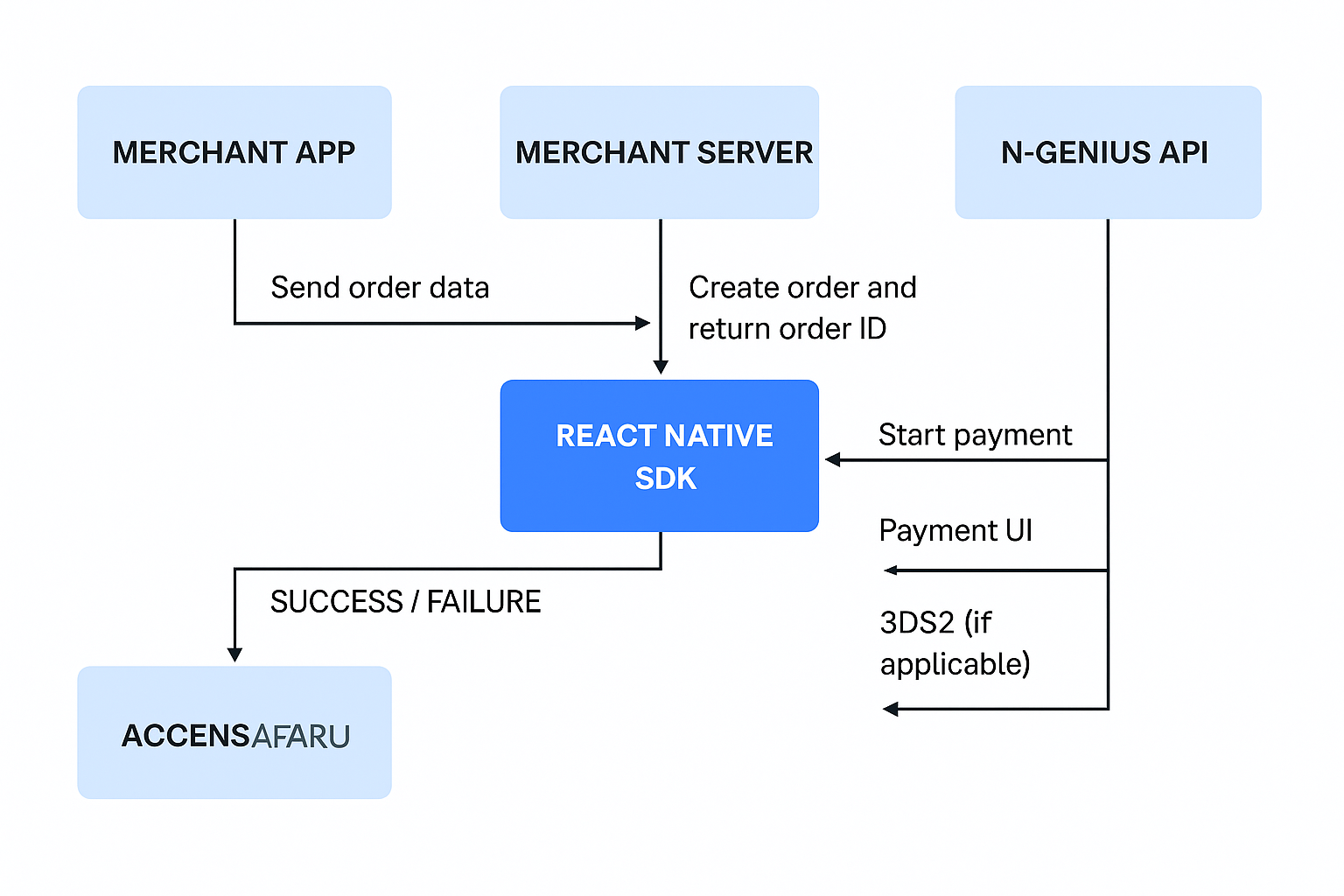
React Native SDK Payment Flow
Installation
You can install the SDK using either npm or yarn:
npm install @network-international/react-native-payment-sdk
Or:
yarn add @network-international/react-native-payment-sdk
For iOS, make sure to run:
cd ios && pod install
If your project targets iOS 10, you must update your deployment target to 11.0+ in your
Podfile
and.xcworkspace
project settings.
To download the React Native repository, click here.
Requirements
Requirement | Details |
---|---|
React Native | 0.60+ |
iOS Deployment Target | 12.0+ (Minimum 11.0) |
Android minSDK Version | 19+ |
Backend Server | Must handle authentication and order creation |
Usage Example
Here is a basic example of initiating a payment:
import { startPayment } from '@network-international/react-native-payment-sdk';
const handlePayment = async () => {
const response = await startPayment({
orderId: 'YOUR_ORDER_ID_HERE',
environment: 'SANDBOX', // or 'PRODUCTION'
});
if (response.status === 'SUCCESS') {
console.log('Payment successful!');
} else {
console.warn('Payment failed:', response.errorMessage);
}
};
Supported Payment Methods
Card Payment
import { initiateCardPayment } from '@network-international/react-native-payment-sdk';
const makeCardPayment = async () => {
try {
const resp = await initiateCardPayment(order);
} catch (err) {
console.log({ err });
}
};
Samsung Pay
import { initiateSamsungPay } from '@network-international/react-native-payment-sdk';
const makeSamsungPayPayment = async () => {
try {
const resp = await initiateSamsungPay(order, merchantName, serviceId);
} catch (err) {
console.log({ err });
}
};
Apple Pay
import { initiateApplePay } from '@network-international/react-native-payment-sdk';
const makeApplePayPayment = async () => {
try {
const resp = await initiateApplePay(order, {
merchantIdentifier: '', // From Apple portal
countryCode: '', // e.g. 'AE' for UAE
merchantName: '', // Displayed on Apple Pay button
});
} catch (err) {
console.log({ err });
}
};
SDK Configuration
Set Display Language
import { configureSDK } from '@network-international/react-native-payment-sdk';
configureSDK({
language: isEnglish ? 'en' : 'ar',
});
Show Order Amount
configureSDK({
shouldShowOrderAmount: true,
});
Checking Payment Support
Samsung Pay Support
import { isSamsungPaySupported } from '@network-international/react-native-payment-sdk';
const isSamsungPayEnabled = await isSamsungPaySupported();
Apple Pay Support
import { isApplePaySupported } from '@network-international/react-native-payment-sdk';
const isApplePayEnabled = await isApplePaySupported();
Features and Platform Support
Feature | Android | iOS |
---|---|---|
initiateCardPayment | ✅ | ✅ |
initiateSamsungPay | ✅ | 🚫 |
initiateApplePay | 🚫 | ✅ |
configureSDK - shouldShowOrderAmount | ✅ | 🚫 |
configureSDK - language | 🚫 | ✅ |
Integration Flow
- The app collects order details.
- These details are sent to your merchant server.
- Your server creates an order via the N-Genius API and returns the
orderId
. - The React Native app uses this
orderId
to initiate the payment. - The SDK handles the payment UI.
- A success or failure callback is returned to the app.
View flowchart: React Native SDK Flowchart (Add image URL once uploaded)
Handling Results
The SDK returns a structured response after the transaction is completed:
{
"status": "SUCCESS", // or "FAILURE"
"transactionId": "123456789",
"errorMessage": "" // present only if status is FAILURE
}
Use this response to update the UI, notify the user, or trigger backend updates.
3DS2 Support for Saved Cards
To invoke the EMV 3-D Secure workflow (commonly used with saved cards), use the executeThreeDSTwo
method:
executeThreeDSTwo(paymentResponse)
Where paymentResponse
is the full JSON response returned by N-Genius to your merchant server when using the savedCard
payment request API.
Need help preparing the
paymentResponse
and handling saved card payments? See the details recipe below:
Testing
Use your UAT credentials and one of the following test cards to simulate payment:
- Card Number: 4111 1111 1111 1111
- Expiry Date: Any future date
- CVV: Any 3-digit number
Test cards will not result in real charges.
Additional Resources
If you encounter issues, contact our support team.